Display a Task List
Chapter Objectives
- ✔️Discover the *ngFor structural directiveLearn how to iterate over a list of items in an HTML Template using the *ngFor directive.
HTML Structure
To display an array of elements, the expected HTML structure is:
<table class="table"> <thead> <tr> <th>Name</th> <th>Date</th> <th>Actions</th> </tr> </thead> <tbody> <tr> <td>Follow the course</td> <td>05/24/2024</td> <td></td> </tr> <tr> <td>Follow the course</td> <td>05/24/2024</td> <td></td> </tr> </tbody></table>
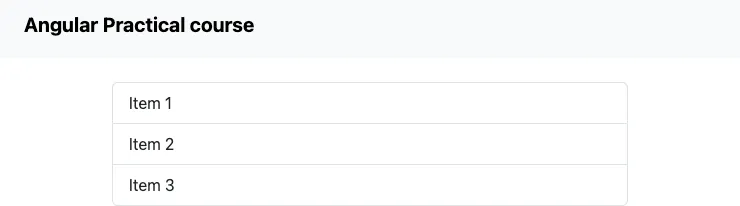
However, what happens if you want to display a dynamic array of elements that changes over time? What if a new task is added?
You’ll want to rely on the tasks variable to display the correct list of tasks. If the tasks are updated, the view (HTML Template) should be updated as well.
Angular Directives
Angular Components are Angular Directives that have an HTML file. The purpose of a Directive is not to display content but to manipulate the HTML Template of another Angular component. They can help dynamically hide/show certain content, modify their style, or affect how many times content is displayed.
*ngFor Structural Directive
The name *ngFor refers to for loop: its purpose is to display a given HTML tag as many times as there are elements in a provided list. You can apply it to any HTML tag. It will affect that tag and its children.
To use it, we add this *ngFor on the HTML tag. It expects an arbitrary syntax that defines both the list and a reference to each list item.
This syntax is let task of tasks
:
-
tasks refers to the list defined in your
component.ts
file. -
task is the reference to use the value of each list item (you can name it whatever you want).
<table class="table"><thead><tr><th>Name</th><th>Date</th><th>Actions</th></tr></thead><tbody><tr *ngFor="let task of tasks"><td>Follow the course</td><td>05/24/2024</td><td></td></tr></tbody></table>
🎓 Instructions
-
Modify the
src/app/task-list.component.html
file.task-list.component.html <table class="table"><thead><tr><th scope="col">Name</th><th scope="col">Date</th><th scope="col">Actions</th></tr></thead><tbody><tr *ngFor="let task of tasks"><td>Follow the course</td><td>05/24/2024</td><td></td></tr></tbody></table> -
Go back to your browser and check the following page:
Your list is now displayed in the browser.
You should see Task
repeated as many times (2) as there are tasks in the list.
✔️ What you’ve learned
In this chapter, you learned how to use a list of elements defined in your component.ts
file in the component.html
file.
You used the *ngFor structural directive to iterate through the list of elements and display them in the view (HTML Template).
Such an action is quite common in Web development, and you’ll likely use it in several of your Angular applications.